Angular UI Components Documentation
Angular CLI with jQWidgets Grid
This tutorial will show you how to use Angular CLI along with the jQWidgets Angular Grid.
Please, follow the instructions below:
First we need to install the Angular CLI globally, so we can have it's commands available:
npm install -g @angular/cli
NOTE: If you run the command from command prompt, make sure you run it as administrator.
Create an Angular CLI application:
ng new jqwidgets-angular-cli-app
NOTE: Node.js version
After running "ng new jqwidgets-angular-cli-app".
If you have an older version of Node.js installed, you may get the following message:
You are running version v6.9.2 of Node.js, which is not supported by Angular CLI v6.
The official Node.js version that is supported is 8.9 and greater.
Please visit https://nodejs.org/en/ to find instructions on how to update Node.js.
- To resolve it, download the latest version of Node.js from https://nodejs.org/en/. Install it and run the command again.
Navigate to the application:
cd jqwidgets-angular-cli-app
Install the jQWidgets dependency:
npm install jqwidgets-scripts --save
By now we have a fully working Angular CLI app. But we need to modify it abit so we can use the jQWidgets Angular Grid. Open the angular.json file and inside the styles property add this line:
"node_modules/jqwidgets-scripts/jqwidgets/styles/jqx.base.css"
It should look like this:

Now open the tsconfig.json file and add this at the bottom:
"include": [
"src/**/*"
],
"files": [
"node_modules/jqwidgets-scripts/jqwidgets-ts/angular_jqxgrid.ts"
]
It should look like this:
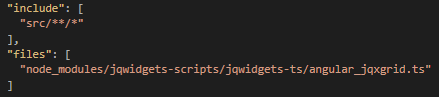
{
"compileOnSave": false,
"compilerOptions": {
"baseUrl": "./",
"outDir": "./dist/out-tsc",
"sourceMap": true,
"declaration": false,
"moduleResolution": "node",
"emitDecoratorMetadata": true,
"experimentalDecorators": true,
"target": "es5",
"typeRoots": [
"node_modules/@types"
],
"lib": [
"es2017",
"dom"
]
},
"include": [
"src/**/*"
],
"files": [
"node_modules/jqwidgets-scripts/jqwidgets-ts/angular_jqxgrid.ts"
]
}
In the files section, we have the jqxGrid typescript file included. This is for the current example. We should add the Typescript files for each widget we are using.
Now when the configuration is done, time to write the application logic itself.
Navigate to src/app and edit the files:
app.module.ts
import { NgModule } from '@angular/core';import { BrowserModule } from '@angular/platform-browser'; import { AppComponent } from './app.component';import { jqxGridComponent } from 'jqwidgets-scripts/jqwidgets-ts/angular_jqxgrid'; @NgModule({ declarations: [ AppComponent, jqxGridComponent ], imports: [ BrowserModule ], bootstrap: [ AppComponent ]}) export class AppModule {}
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css']}) export class AppComponent { source: any = { localdata: [ ['Alfreds Futterkiste', 'Maria Anders', 'Sales Representative', 'Obere Str. 57', 'Berlin', 'Germany'], ['Ana Trujillo Emparedados y helados', 'Ana Trujillo', 'Owner', 'Avda. de la Constitucin 2222', 'Mxico D.F.', 'Mexico'], ['Antonio Moreno Taquera', 'Antonio Moreno', 'Owner', 'Mataderos 2312', 'Mxico D.F.', 'Mexico'], ['Around the Horn', 'Thomas Hardy', 'Sales Representative', '120 Hanover Sq.', 'London', 'UK'], ['Berglunds snabbkp', 'Christina Berglund', 'Order Administrator', 'Berguvsvgen 8', 'Lule', 'Sweden'], ['Blauer See Delikatessen', 'Hanna Moos', 'Sales Representative', 'Forsterstr. 57', 'Mannheim', 'Germany'], ['Blondesddsl pre et fils', 'Frdrique Citeaux', 'Marketing Manager', '24, place Klber', 'Strasbourg', 'France'], ['Blido Comidas preparadas', 'Martn Sommer', 'Owner', 'C\/ Araquil, 67', 'Madrid', 'Spain'], ['Bon app', 'Laurence Lebihan', 'Owner', '12, rue des Bouchers', 'Marseille', 'France'], ['Bottom-Dollar Markets', 'Elizabeth Lincoln', 'Accounting Manager', '23 Tsawassen Blvd.', 'Tsawassen', 'Canada'], ['B`s Beverages', 'Victoria Ashworth', 'Sales Representative', 'Fauntleroy Circus', 'London', 'UK'], ['Cactus Comidas para llelet', 'Patricio Simpson', 'Sales Agent', 'Cerrito 333', 'Buenos Aires', 'Argentina'], ['Centro comercial Moctezuma', 'Francisco Chang', 'Marketing Manager', 'Sierras de Granada 9993', 'Mxico D.F.', 'Mexico'], ['Chop-suey Chinese', 'Yang Wang', 'Owner', 'Hauptstr. 29', 'Bern', 'Switzerland'], ['Comrcio Mineiro', 'Pedro Afonso', 'Sales Associate', 'Av. dos Lusadas, 23', 'Sao Paulo', 'Brazil'], ['Consolidated Holdings', 'Elizabeth Brown', 'Sales Representative', 'Berkeley Gardens 12 Brewery', 'London', 'UK'], ['Drachenblut Delikatessen', 'Sven Ottlieb', 'Order Administrator', 'Walserweg 21', 'Aachen', 'Germany'], ['Du monde entier', 'Janine Labrune', 'Owner', '67, rue des Cinquante Otages', 'Nantes', 'France'], ['Eastern Connection', 'Ann Devon', 'Sales Agent', '35 King George', 'London', 'UK'], ['Ernst Handel', 'Roland Mendel', 'Sales Manager', 'Kirchgasse 6', 'Graz', 'Austria'] ], datafields: [ { name: 'CompanyName', type: 'string', map: '0' }, { name: 'ContactName', type: 'string', map: '1' }, { name: 'Title', type: 'string', map: '2' }, { name: 'Address', type: 'string', map: '3' }, { name: 'City', type: 'string', map: '4' }, { name: 'Country', type: 'string', map: '5' } ], datatype: 'array' }; dataAdapter: any = new jqx.dataAdapter(this.source); columns: any[] = [ { text: 'Company Name', datafield: 'CompanyName', width: 200 }, { text: 'Contact Name', datafield: 'ContactName', width: 150 }, { text: 'Contact Title', datafield: 'Title', width: 100 }, { text: 'Address', datafield: 'Address', width: 100 }, { text: 'City', datafield: 'City', width: 100 }, { text: 'Country', datafield: 'Country' } ];}
app.component.html
<jqxGrid [width]="850" [source]="dataAdapter" [columns]="columns" [columnsresize]="true" [sortable]="true"></jqxGrid>
Time has come to run the application. In the command line type:
ng serve

That can be resolved in two ways:
Add "src/app/app.module.ts", in the ‘files’ array in the tsconfig.json file":
"files": [
"src/app/app.module.ts",
"node_modules/jqwidgets-scripts/jqwidgets-ts/angular_jqxbargauge.ts"
]
The second way is: Go to src/app/app.module.ts, remove a random line, save the file, add back the line and run ng serve again.
Navigate to localhost:4200 and see the result:
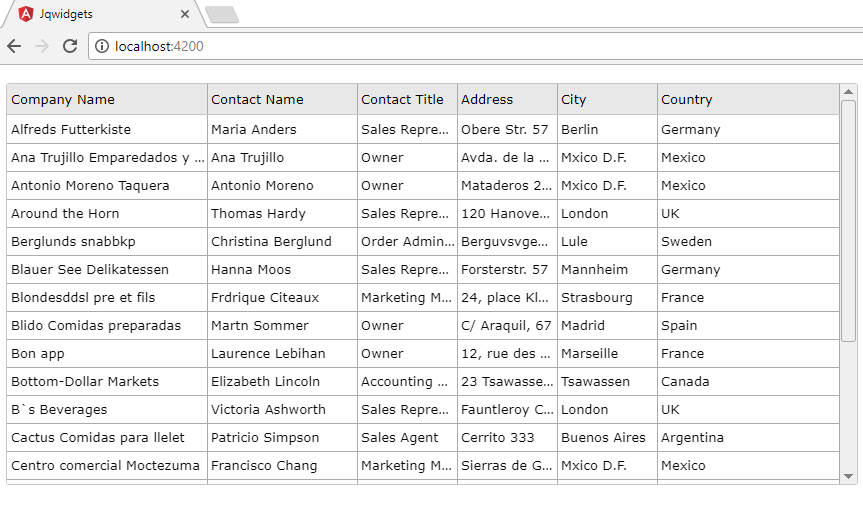
A video tutorial is also available on YouTube: Tutorial: Fast and easy way to integrate jqxGrid in Angular project